Singleton Pattern
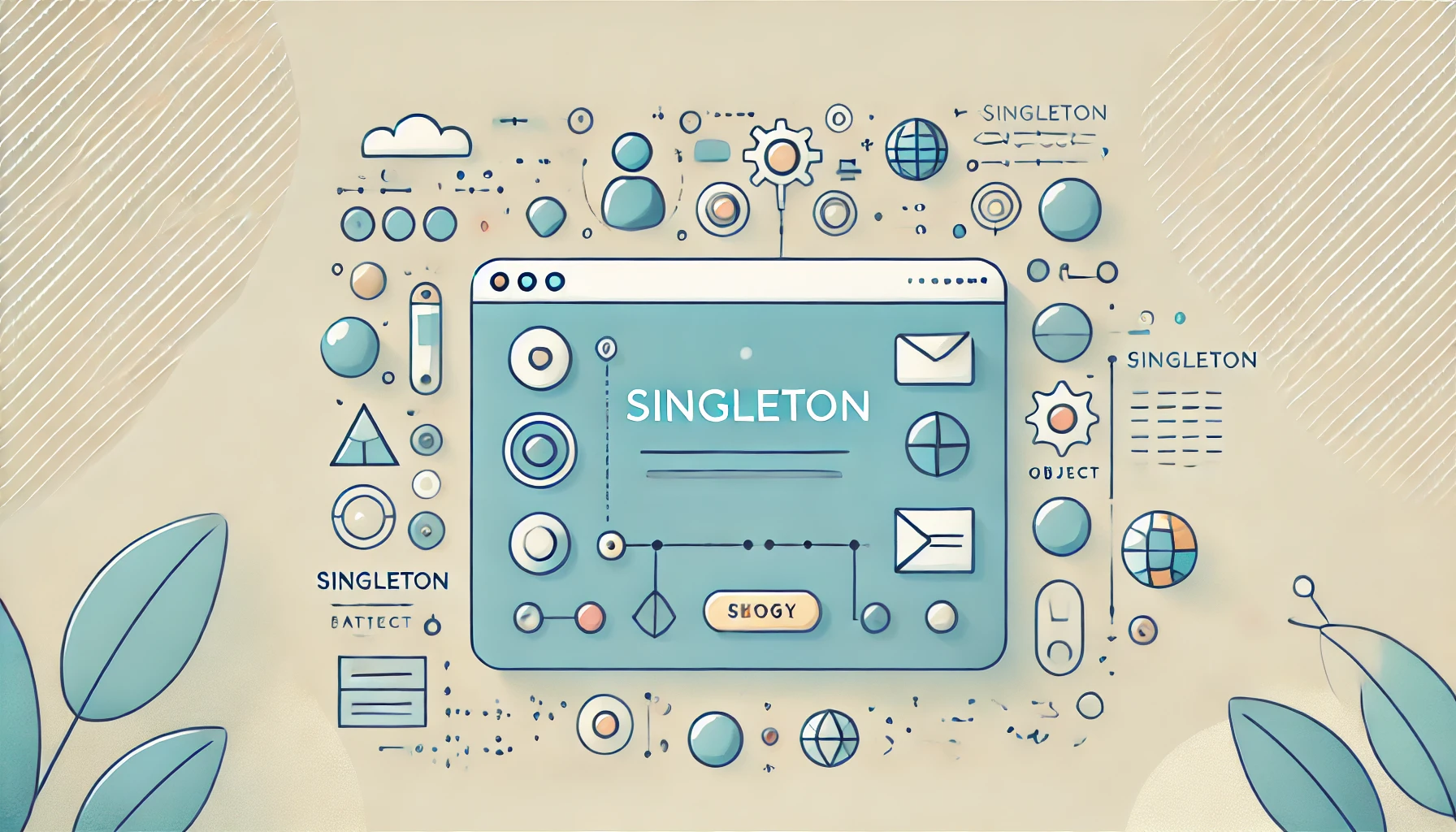
Singleton Pattern is a creational design pattern that makes sure that only a single instance is created for a class. It also provides global access to this single instance so that anyone can use the same instance.
Structure
The Singleton pattern consists of only a single class.
- It contains the
getInstance
method which returns the only instance of the class - The default constructor is set as private so that no one else apart from the getInstance method can access it
When to use the Singleton Pattern?
- When you want a class in your application only to have a single instance (database connection, etc.)
- When you want to put access control on global variables
Something to remember is that using global variables is considered a bad coding practice, and singleton pattern use global variables
Singleton pattern also violates the
Single Responsibility Principle
, it controls the object creation and can also contain some business logic
Implementation Example
The code below demonstrates the implementation of the Singleton design pattern in Java. The DatabaseConnection is a singleton and would always return the same single instance, which can be verified by trying to initialize two separate instances.
// client class
public class Solution {
public static void main(String[] args) {
DatabaseConnection connection1 = DatabaseConnection.getInstance();
DatabaseConnection connection2 = DatabaseConnection.getInstance();
System.out.println("Two objects are the same: " + connection1.equals(connection2));
System.out.println("Connection 1: " + connection1.toString() + "\nConnection 2: " + connection2.toString());
}
}
// the singleton class which would only have a single instance
class DatabaseConnection {
private static DatabaseConnection databaseConnection;
private DatabaseConnection() {
}
// method to return the only instance of the class
public static DatabaseConnection getInstance() {
if (databaseConnection == null) {
databaseConnection = new DatabaseConnection();
}
return databaseConnection;
}
}
The output for the following program will be:
Two connections are same: true
Connection 1: DatabaseConnection@58644d46
Connection 2: DatabaseConnection@58644d46
This is the last design pattern in the Creational Patterns category, we will look at Structural Patterns in further blogs.
Enjoyed the read? Give it a thumbs up 👍🏻!