Hello World AWS CDK App
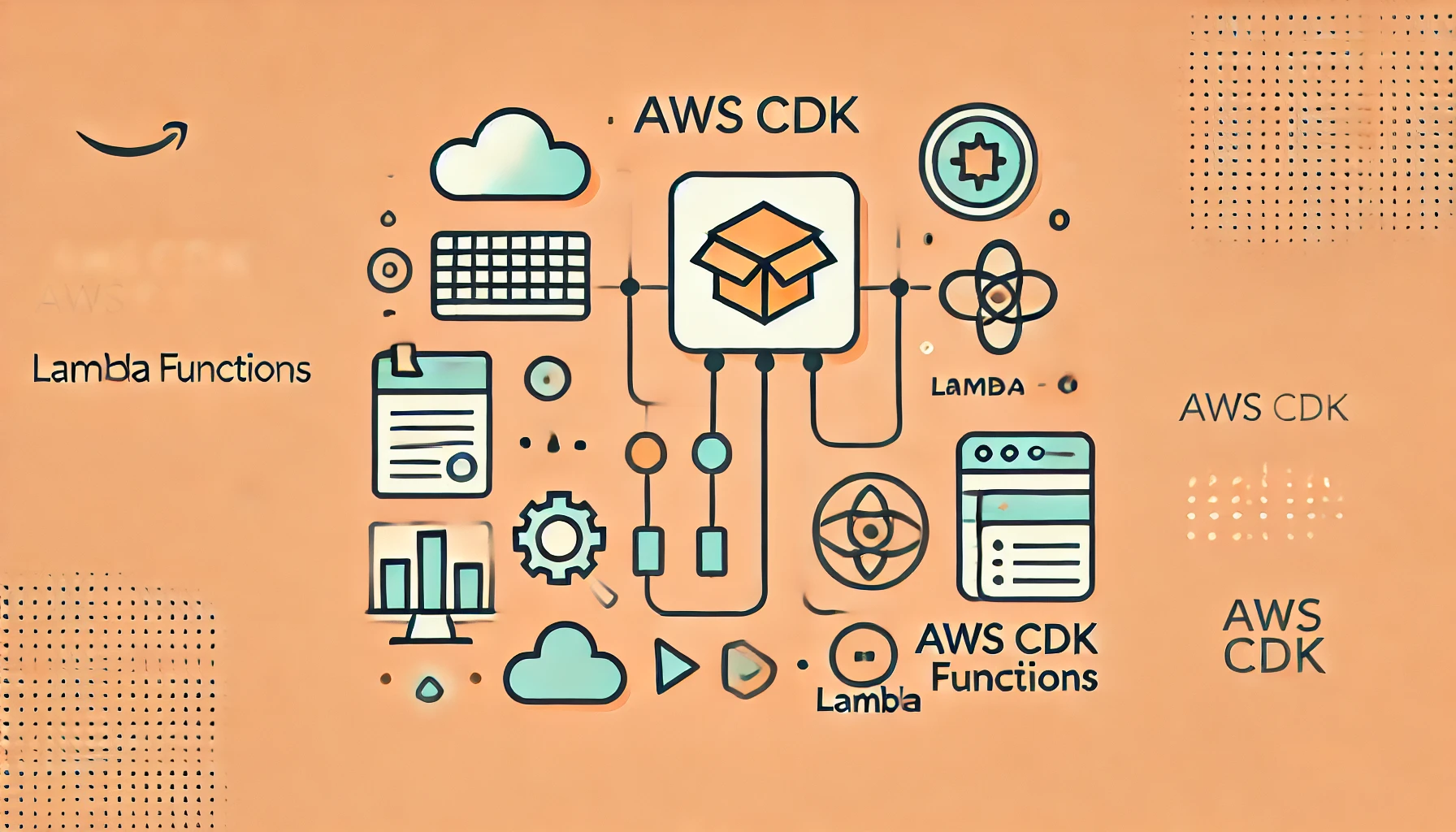
The AWS Cloud Development Kit (AWS CDK) is an open-source software development framework that allows us to define Infrastructure as Code (IaC), and provision the resources using AWS CloudFormation. The AWS CDK supports TypeScript, JavaScript, Python, Java, C#/.Net, and Go for writing CDK apps.
In this tutorial, we will create and deploy a simple AWS CDK app containing one AWS Lambda Function which can be accessed using the API Gateway.
Step 1: Create the CDK app
First, create a new directory for the CDK app using the commands given below:
$ mkdir cdk-hello-world
$ cd cdk-hello-world
Next, we use the cdk init
command to initialize the app. We also specify the programming language with the --language
option which we will use in our CDK app. This will initialize a Git repository (if the system has git initialized), and also create some files and folders to organize the source code for the app.
$ cdk init app --language typescript
Step 2: Build the app
We use the build command to build the CDK app in order to catch any syntax or type errors present in the app.
$ npm run build
Step 3: Define Lambda Function Resource
We have a single stack in our CDK app as of now. We will define an Amazon Lambda Function resource within the stack using code. Below is the code which can be used to do so:
// Define the lambda function
const helloWorldFunction = new lambda.Function(this, 'HelloWorldFunction', {
runtime: lambda.Runtime.NODEJS_20_X, // the runtime for the lambda function
code: lambda.Code.fromAsset('lambda'), // source code the the lambda function
handler: 'hello.handler', // handler function for the lambda function
});
Now, we will create a new directory lambda
inside the app directory and a new file hello.js
inside the directory. In the file, we will define the handler function for lambda, which will return a response as shown below.
exports.handler = async (event) => {
return {
statusCode: 200,
headers: { "Content-Type": "text/plain" },
body: JSON.stringify({ message: "Hello, World!" }),
};
};
Step 4: Defile API Gateway REST API Resource
To define API Gateway, we import the aws-apigateway
construct from the AWS library. Here, we will define the lambda function created in Step 3 as the handler for the API Gateway.
// Define the API Gateway resource
const api = new apigateway.LambdaRestApi(this, 'HelloWorldApi', {
handler: helloWorldFunction,
proxy: false,
});
Next, we will add a path named hello
to the root of the API endpoint created above. This will create a new endpoint that adds /hello
to the base URL. Finally, we add a GET method to the hello resource.
// Define the '/hello' resource with a GET method
const helloResource = api.root.addResource('hello');
helloResource.addMethod('GET');
As of now, when our /hello
endpoint will receive a GET request the lambda function will be invoked which will return the response defined in the hello.js
file.
Step 5: Build and Synthesize the CDK app
Build the app:
$ npm run build
Synthesize an AS CloudFormation template from the CDK code, here you may need to use your currently logged in aws profile using the --profile
option:
$ cdk synth
Step 6: Deploy the CDK application
We use the cdk deploy
command to deploy the application. Confirm the changes when prompted:
$ cdk deploy
Once the app has been deployed, you will be provided with the endpoint URL for the API gateway. You can use this endpoint to make a GET (at /hello) request, and it should return the hello world message.
Step 7: Destroy the CDK application
Run the cdk destroy
command and confirm when prompted.
$ cdk destroy
Note: You might need to specify the CDK profile to use while running all the cdk commands, use your profile with the –profile option in the command (
cdk destroy --profile profile-name
).
References
- AWS CDK Docs
Enjoyed the read? Give it a thumbs up 👍🏻!